Debugging tools
Playwright scripts work with existing debugging tools, like Node.js debuggers and browser developer tools. Playwright also introduces new debugging features for browser automation.
- Run in headful mode
- Visual Studio Code debugger (Node.JS)
- Browser Developer Tools
- Run in Debug Mode
- Verbose API logs
#
Run in headful modePlaywright runs browsers in headless mode by default. To change this behavior, use headless: false
as a launch option. You can also use the slow_mo
option to slow down execution and follow along while debugging.
- Sync
- Async
#
Visual Studio Code debugger (Node.JS)The VS Code debugger can be used to pause and resume execution of Playwright scripts with breakpoints. The debugger can be configured in two ways.
#
Use launch configSetup launch.json
configuration for your Node.js project. Once configured launch the scripts with F5 and use breakpoints.
#
Use the new JavaScript debugging terminalVS Code 1.46+ introduced the new JavaScript debugger that does not require a launch.json
configuration. To use it:
- Set a breakpoint in VS Code
- Use the
debugger
keyword or set a breakpoint in the VS Code UI
- Use the
- Run your Node.js script from the terminal
#
Browser Developer ToolsYou can use browser developer tools in Chromium, Firefox and WebKit while running a Playwright script. Developer tools help to:
- Inspect the DOM tree and find element selectors
- See console logs during execution (or learn how to read logs via API)
- Check network activity and other developer tools features
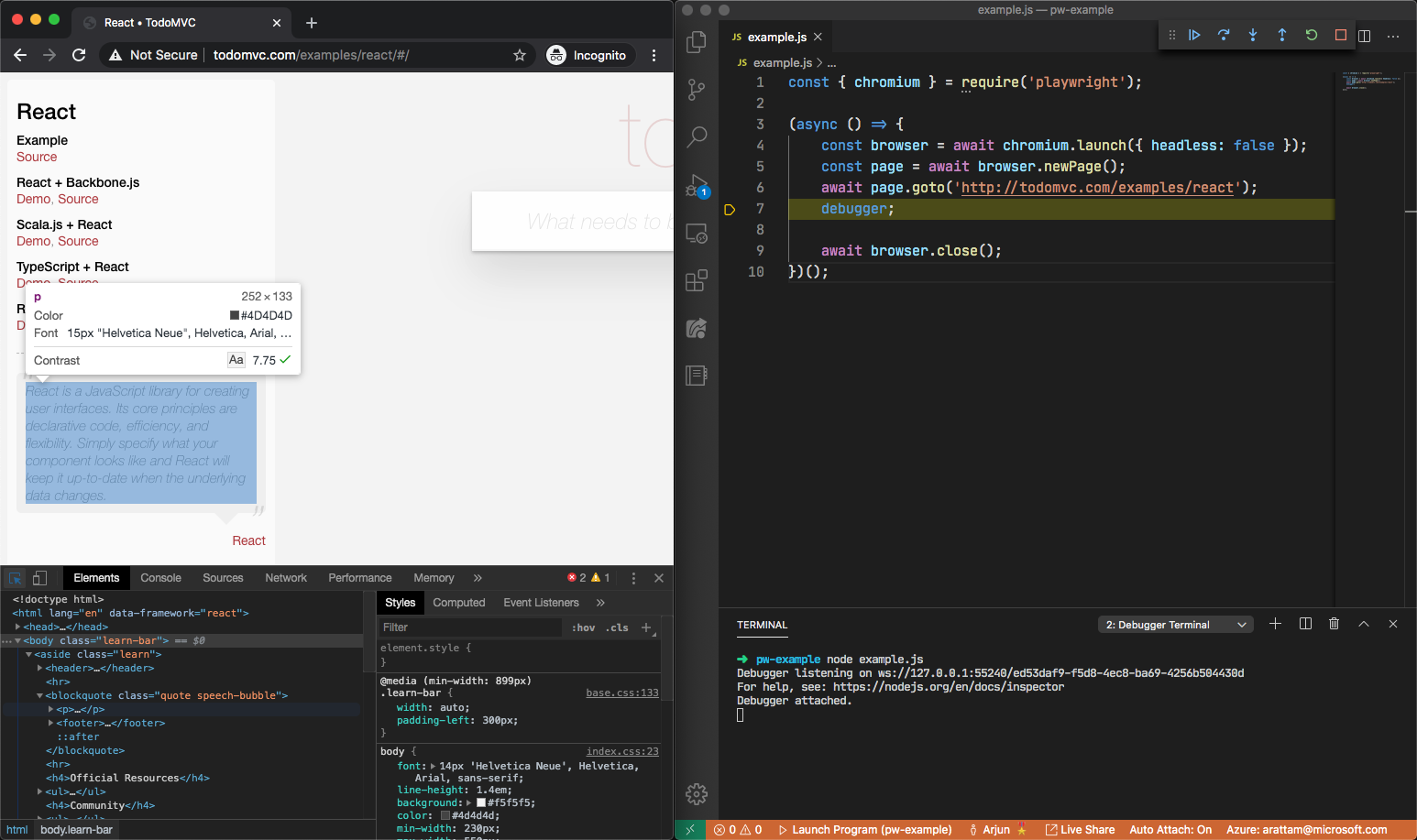
For WebKit: Note that launching WebKit Inspector during the execution will prevent the Playwright script from executing any further.
#
API for ChromiumIn Chromium, you can also open developer tools through a launch option.
- Sync
- Async
#
Run in Debug ModeSet the PWDEBUG
environment variable to run your scripts in debug mode. This configures the browser for debugging.
#
DefaultsWith PWDEBUG, the following defaults are configured for you:
- Run in headful: With PWDEBUG, browsers always launch in headful mode
- Disables timeout: PWDEBUG sets timeout to 0 (= no timeout)
- Preserve DevTools preferences: When used with
devtools: true
, PWDEBUG preserves the docked/undocked state of Chrome DevTools
#
Debugging SelectorsPWDEBUG configures a playwright
object in the browser to highlight Playwright selectors. This can be used to verify text or composite selectors. To use this:
- Setup a breakpoint to pause the execution
- Open the console panel in browser developer tools
- Use the
playwright
APIplaywright.$(selector)
: Highlight the first occurrence of the selector. This reflects howpage.$
would see the page.playwright.$$(selector)
: Highlight all occurrences of the selector. This reflects howpage.$$
would see the page.playwright.inspect(selector)
: Inspect the selector in the Elements panel.playwright.clear()
: Clear existing highlights.
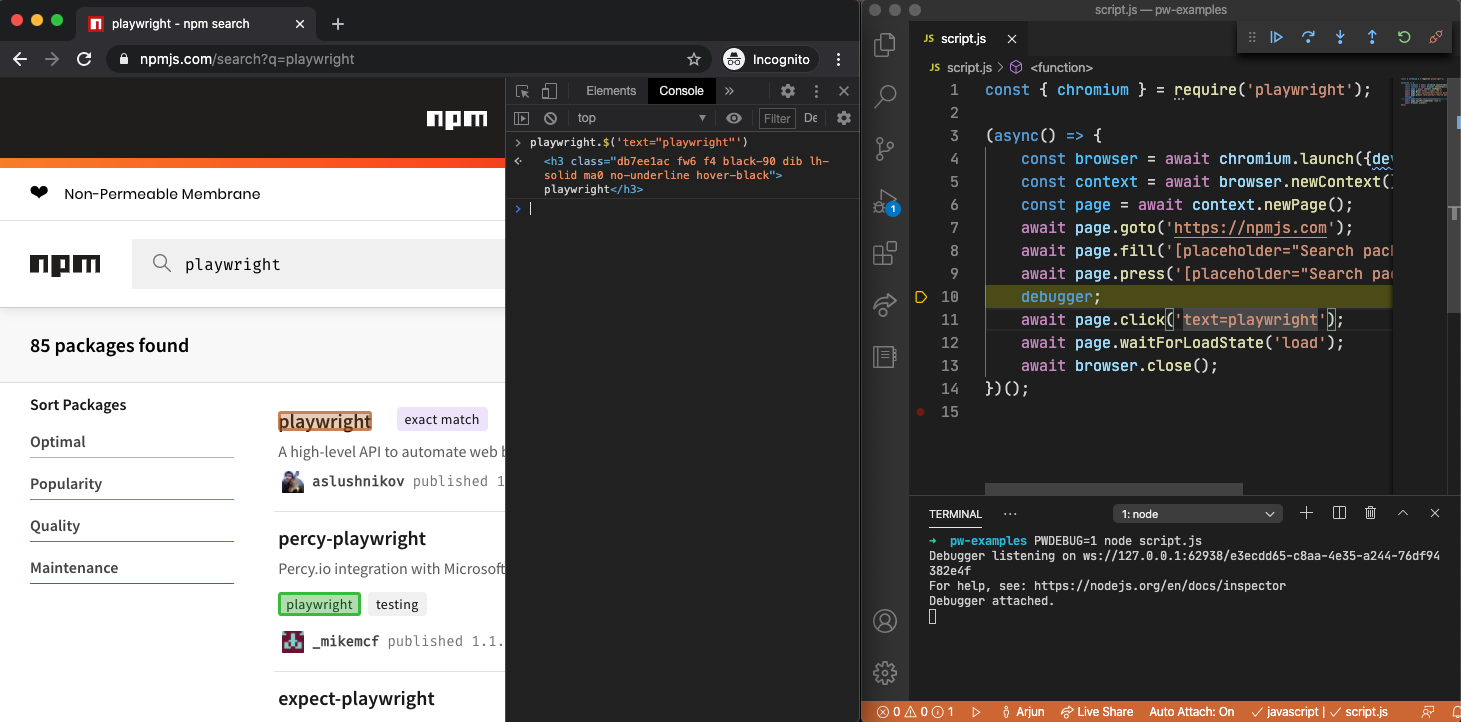
#
Evaluate Source MapsPWDEBUG also enables source maps for page.evaluate(expression, **kwargs) executions. This improves the debugging experience for JavaScript executions in the page context.
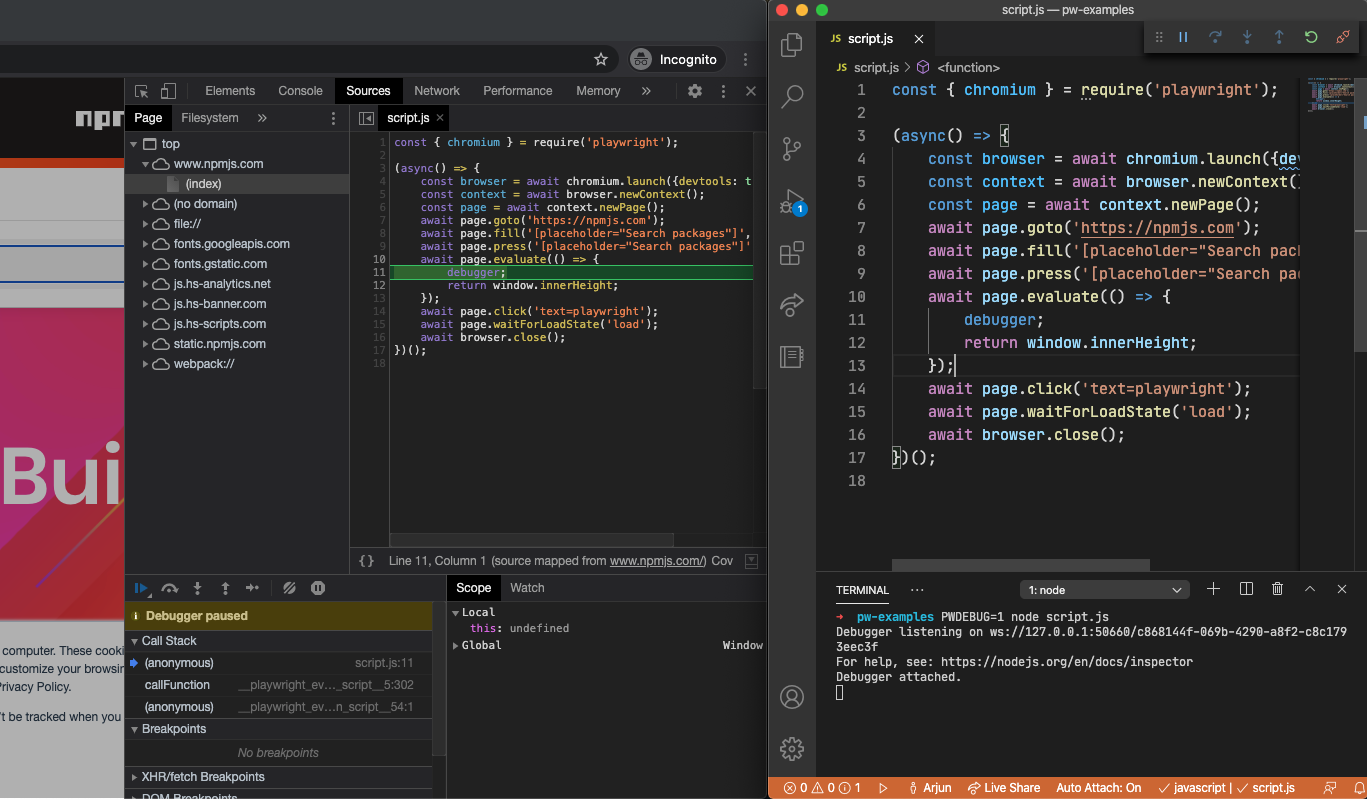
#
Verbose API logsPlaywright supports verbose logging with the DEBUG
environment variable.